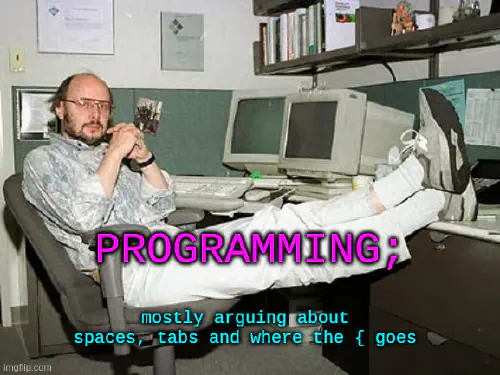
Macro List Pattern
Keep your list of data abstract, format it at compile time!
Modified:
Created:
This is an oldy, but goody. This pattern allows you to make an abstract list of data and then format it at will during compile time. I’m a big proponent of single source of truth (also known as DRY, or don’t repeat yourself). This is one way to achieve this.
Steps
Create your list
Use a macro that is undefined, here that’s X(). Note, here the example has two fields per list item, but you can have N fields as macro input arguments.
c++
#define MY_LIST \
X(Item1, "This is a description of item 1") \
X(Item2, "This is a description of item 2") \
X(Item3, "This is a description of item 3")
// And so on...
Define macro, use the list, undefine macro
Since our X macro is undefined, we can define it for the region of code where we’re using it. This allows us to redefine the macro in different use cases.
c++
// Create an enumeration
#define X(name, desc) name,
enum ListItemType { MY_LIST }
#undef X
// Initialize a description map
#define X(name, desc) { ListItemType::name, desc },
static std::map<ListItemType, String> listItemTypeDesc = { MY_LIST };
#undef X